Showing a convenient way on how to use Secret Manager to securely pass sensible data as environment values to Google App Engine (GAE) services running with Node.js.
Unfortunately, App Engine doesn’t deliver an out-of-the box solution for passing env vars from Secret Manager like it’s available in Cloud Functions by using the --set-secrets
option of gcloud functions deploy
.
In this article a convenient way by using a simple npm package is shown to achieve this. The goals are:
Let’s go to it…
Table of Contents
Integrating Secret Manager with App Engine
Setting-up a secret in GCP Secret Manager
First, create one or more secrets in Secret Manager of your GCP project. Here, the secret is named MY_SECRET
and has a reference path of projects/100374066341/secrets/
MY_SECRET.
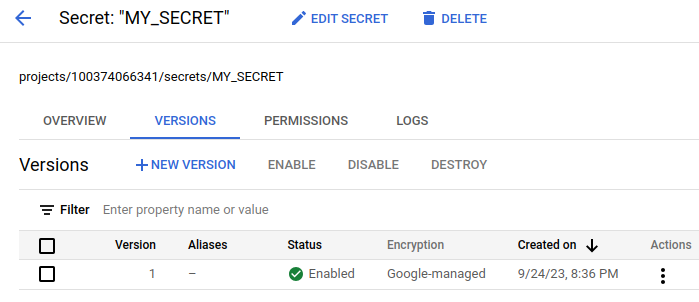
For a more detailed guide on how to enbale Secret Manager and creating secrets please refer to this section about secure configuration management in Cloud Functions.
Granting Secret Manager rights to the GAE service account
In order to resolve secrets from Secret Manager, the service account principal running your App Engine service – by default PROJECT_ID@appspot.gserviceaccount.com
– must have at least the Secret Manager Secret Accessor
role. For more details refer to the Secret Manager access control documentation.
To do so, go to IAM in the console and edit the App Engine principal. There, click “Add another role” and search for Secret Manager Secret Accessor
and save, like so.
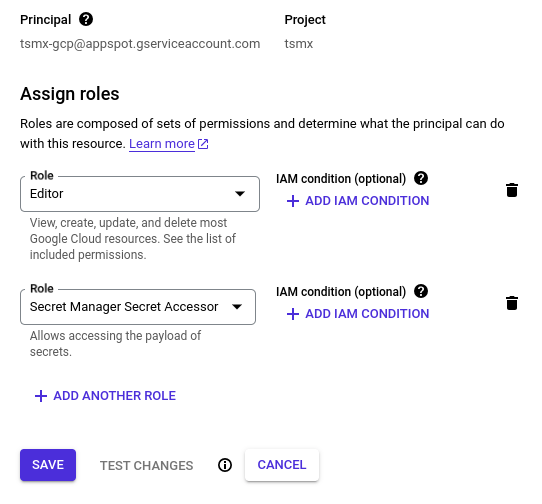
Referencing a secret in app.yaml
In the standard app.yaml
deployment descriptor of your App Engine service, create an appropriate environment variable in the env_variables
section containing the secrets reference path. Like so…
service: my-service
runtime: nodejs20
env_variables:
MY_SECRET: "projects/100374066341/secrets/MY_SECRET/versions/latest"
Note that you have to add /versions/latest
to reference the lastest version of the secret or /versions/x
to reference the version with number x, e.g. /versions/2
. For details see referencing secrets.
Add the gae-env-secrets package to your project
Next, add the gae-env-secrets package as a dependency in your project. This will provide the functionality to retrieve Secret Manager values for environment variables used in App Engine.
npm i gae-env-secrets --save
Use the Secret Manager value in your code
Import the gae-env-secrets
package in your code and call the async getEnvSecrets
function out of it. Once completed, you’ll be able to access the values stored in GCP Secret Manager by simply accessing the env vars used in the deployment descriptor. Works with CommonJS as well as ESM.
CommonJS
const { getEnvSecrets } = require('gae-env-secrets');
getEnvSecrets().then(() => {
const secret = process.env['MY_SECRET']; // value of MY_SECRET from Secret Manager
});
ESM
import { getEnvSecrets } from 'gae-env-secrets';
await getEnvSecrets();
const secret = process.env['MY_SECRET']; // value of MY_SECRET from Secret Manager
That’s it. You can now seamlessly use Secret Manager secret values in your GAE App Engine services by referencing env vars.
To learn more on how the gae-env-secrets
package is working and how its usage can be customized, read on.
Under the hood
Referencing secrets in the deployment descriptor
To reference secrets in the app.yaml
deployment descriptor, you’ll need to pass the versioned reference of the secret from Secret Manager. This has the form of…
projects/[Project-Number]/secrets/[Secret-Name]/versions/[Version-Number|latest]
To retrieve the reference path of a secrets version in Secret Manager simply click “Copy resource name” on the three dots behind a version. Specifying latest
as the version instead of a number will always supply the highest active version of a secret.
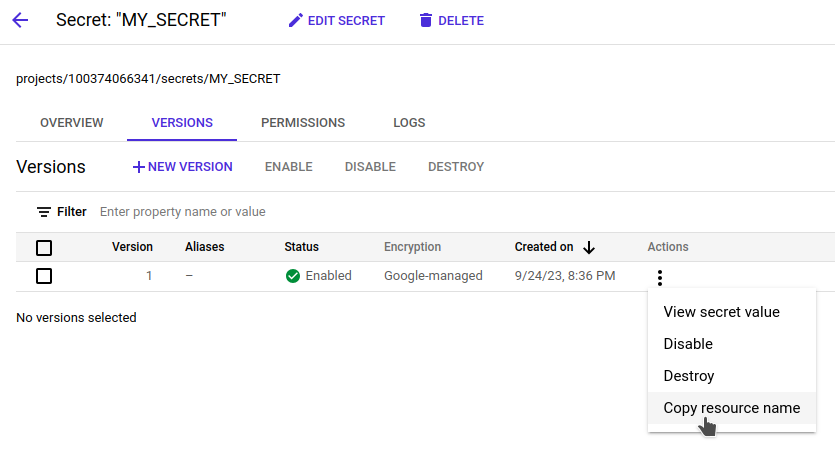
Then pass the secrets reference to the desired variable in the env_variables
block of the deployment descriptor, like so…
env_variables:
SECRET_ENV_VAR: "projects/100374066341/secrets/MY_SECRET/versions/1"
For more details, refer to the app.yaml reference.
Determining the runtime-environment
gae-env-secrets
will evaluate environment variables to detect if it is running directly in App Engine. If the following env vars both are present, the library would assume it’s running in GAE and substitute relevant env vars with their respective secret values from Secret Manager:
GAE_SERVICE
GAE_RUNTIME
If these two env vars are not present, the library won’t do anything. So it should be safe to call it unconditionally in your code without inferring local development, testing etc.
To simulate running under GAE, simply set those two env vars to anything.
Substituting env vars from Secret Manager
If running under GAE is detected, calling getEnvSecrets
will iterate through all env vars and substitute the value with the corresponding secret derived from Secret Manager if one of the following condition is true:
- The name of the env var ends with
_SECRET
(default suffix) or another deviating suffix passed via the options - Auto-Detection is enabled via options and the value of the anv var matches a Secret Manager secret reference
For accessing the Secret Manager, the library uses the package @google-cloud/secret-manager.
Error handling
By default and for security reasons, the library will throw
an error if substituting an env vars value from Secret Manager fails for any reason…
- secret reference is invalid
- secret is inactive or not present
- invalid version number
- missing permissions to access Secret Manager
- or else…
So make sure to use an appropriate error handling with try/catch
or .catch()
.
To change this behaviour, use the strict property available in the options.
Passing options to getEnvSecrets
You can pass an options object when calling getEnvSecrets
to customize the behaviour. The following options are available.
suffix
Type: String
Default: _SECRET
All env vars whose name is ending with the suffix
will be substituted with secrets from Secret Manager.
Pass another value to change the env vars of your choice.
// will substitue all env vars ending with '_KEY'
getEnvSecrets({ suffix: '_KEY' });
strict
Type: Boolean
Default: true
By default strict
is true
which means that if a secret cannot be resolved an error will be thrown.
Setting strict
to false
will change this behaviour so that the error is only written to console.error
. The value of the env var(s) where the error occured will remain unchanged.
// error will only be logged and respective env vars remain unchanged
getEnvSecrets({ strict: false });
autoDetect
Type: Boolean
Default: false
The autoDetect
feature enables automatic detection of env var values that contain a Secret Manager secret reference for substitution regardless of the suffix and env vars name.
This feature is additional to the provided suffix, meaning that all env vars ending with the suffix AND all automatically detected will be substituted.
To turn on this feature, pass true
in the options object.
// turn on autoDetect
getEnvSecret({ autoDetect: true });
Example: Having this feature enabled, the following env var would be substituted with version 2 of the secret MY_SECRET
regardless of the suffix because is contains a value of a Secret Manager reference.
env_variables:
VAR_WITH_ANY_NAME: "projects/00112233/secrets/MY_SECRET/versions/2"
Considerations & limitations when using gae-env-secrets
Please keep in mind the following points when using this solution.
- Since the
getEnvSecrets
function is async you’ll need toawait
the result or chain on using.then
to be able to work with the secret values. CommonJS does not support top-level await. - As the env var secrets are resolved at runtime of your code, any top-level code of other modules that is executed upon
require/import
cannot make use of the secret values and instead would see the secret references as values of the env vars. - Resolving the secrets from Secret Manager using the underlying Google library will usually take 1-2 seconds.
Summary
This article shows how to integrate Secret Manager easily with App Engine by using one simple package and few lines of code. No vendor or platform lock-in is created.
However, once Google is supplying an out-of-the box feature to make the integration work like in Cloud Functions, it should be considered switching to this to maybe overcome the limitations of this solution, e.g. secret resolution at runtime.
Happy coding 🙂